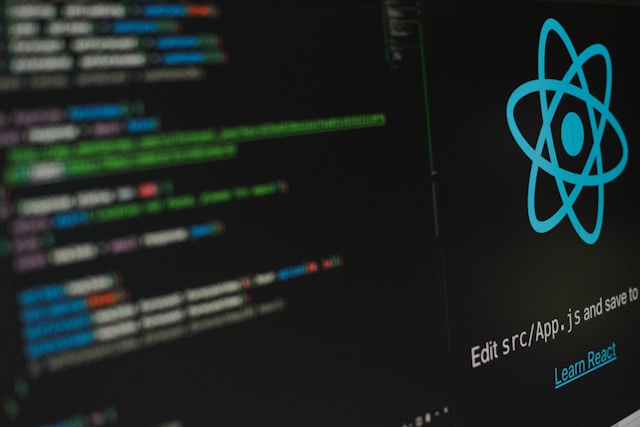
Intro to React: A Beginner’s Guide
Radi Rusadi / September 20, 2024
Introduction
React is a popular JavaScript library for building user interfaces. Created by Facebook, it’s widely used due to its component-based, declarative, and state-driven nature.
-
Component-based: UI is broken down into small, reusable pieces like headers, footers, and buttons.
-
Declarative: React uses JSX syntax, combining HTML, CSS, and JavaScript to define the UI based on data and state.
-
State-driven: React automatically updates the UI when data (state) changes.
Is React a Library or Framework?
React is a library focused only on the “view” layer. For building full applications, developers need additional tools like routing or state management libraries.
Starting a React Project
Here are popular tools to start a React project:
- Create React App (CRA): Recommended for beginners, but for production, it's suggested to use frameworks like Next.js.
- Vite: A fast, modern alternative with built-in TypeScript and ESLint support.
- Next.js: A React framework with advanced features like SEO optimization, server-side rendering, and routing. Great for building full-stack apps.
Other React-based tools include Remix and Gatsby.
React vs. Vanilla JavaScript
Below is a comparison of traditional VanillaJS and React.
VanillaJS Example:
<body>
<div class="container">
<div id="value">0</div>
<div>
<button id="more">+</button>
<button id="less">-</button>
</div>
</div>
</body>
<script>
const value = document.querySelector('#value');
const more = document.querySelector('#more');
const less = document.querySelector('#less');
const incrementHandler = () => {
value.textContent = +value.textContent + 1;
};
const decrementHandler = () => {
value.textContent = +value.textContent - 1;
};
more.addEventListener('click', incrementHandler);
less.addEventListener('click', decrementHandler);
</script>
ReactJS Example:
const Counter = () => {
const [counter, setCounter] = useState(0);
return (
<div className='container'>
<div>{counter}</div>
<div>
<button onClick={()=> setCounter(counter + 1)}>+</button>
<button onClick={()=> setCounter(counter - 1)}>-</button>
</div>
</div>
);
};
As shown, React code is more concise, reusable, and easier to manage, especially for larger applications.
ReactJS vs. VanillaJS: Key Differences
-
UI Building:
- ReactJS: Reusable components.
- VanillaJS: Repeated DOM manipulation.
-
DOM Manipulation:
- ReactJS: Uses a virtual DOM for better performance.
- VanillaJS: Direct DOM access can slow down larger applications.
-
State Management:
- ReactJS: State updates automatically trigger UI changes.
- VanillaJS: Requires manual tracking and updates.
-
Learning Curve:
- ReactJS: Requires learning JSX, hooks, and React-specific tools.
- VanillaJS: Easier to start but harder to scale.
-
Ecosystem:
- ReactJS: Rich ecosystem with built-in tools.
- VanillaJS: Requires custom solutions or third-party libraries.
Summary
ReactJS offers a modern, efficient way to build dynamic, scalable web apps, especially compared to VanillaJS, which can become cumbersome for larger projects. It excels with reusable components, state management, and a rich ecosystem, making it ideal for more complex applications.